Designing the Sign-Up Page for the Flutter Ecommerce App
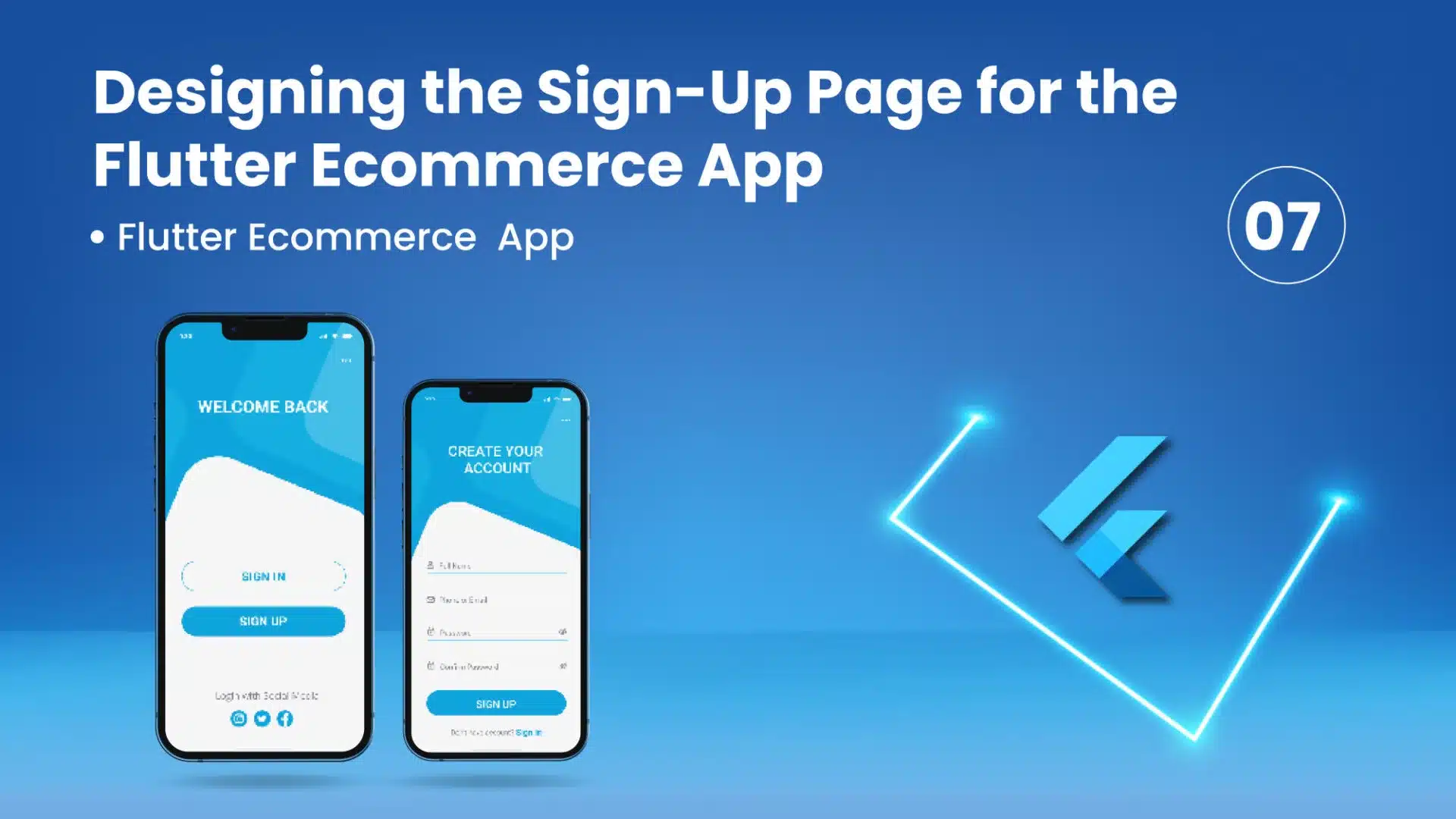
26 July
In this section, we will shift our focus to designing the sign-up page for our Flutter ecommerce app. The sign-up page allows new users to create an account and join the platform. We’ll create a user-friendly sign-up page with input fields for personal info, email, password, and a sign-up button for a seamless experience. Additionally, we may include optional fields for additional details or preferences. Following the principles of responsive design, we will ensure that the page layout adapts seamlessly to various screen sizes. Crafting an enticing, efficient sign-up page, our aim is to provide new users with a seamless onboarding experience. Let’s proceed with designing the sign-up page to facilitate user registration and engagement in our app.
import 'package:clickmart/view/authentication/sign_in.dart'; import 'package:flutter/material.dart'; import 'package:flutter_signin_button/flutter_signin_button.dart'; class SignUp extends StatefulWidget { const SignUp({super.key}); @override State<SignUp> createState() => _SignUpState(); } class _SignUpState extends State<SignUp> { // Form Key final GlobalKey<FormState> _formKey = GlobalKey<FormState>(); // TextForm Controller TextEditingController emailController = TextEditingController(); TextEditingController passwordController = TextEditingController(); // Form Validation bool _validateAndSave() { final form = _formKey.currentState; if (form!.validate()) { form.save(); return true; } return false; } void _validateAndSubmit() { if (_validateAndSave()) { // TODO: Perform sign-up logic here } } @override Widget build(BuildContext context) { return WillPopScope( onWillPop: () { return Future.value(false); }, child: Scaffold( appBar: AppBar( automaticallyImplyLeading: false, title: const Text('Sign Up'), ), body: Container( padding: const EdgeInsets.all(16.0), child: Form( key: _formKey, child: Column( crossAxisAlignment: CrossAxisAlignment.stretch, children: <Widget>[ TextFormField( controller: emailController, decoration: const InputDecoration(labelText: 'Email'), validator: (value) { if (value!.isEmpty) { return 'Please enter your email'; } return null; }, onSaved: (value) => emailController.text = value!, ), const SizedBox(height: 16.0), TextFormField( controller: passwordController, decoration: const InputDecoration(labelText: 'Password'), obscureText: true, validator: (value) { if (value!.isEmpty) { return 'Please enter your password'; } return null; }, onSaved: (value) => passwordController.text = value!, ), const SizedBox(height: 16.0), ElevatedButton( onPressed: _validateAndSubmit, child: const Text('Sign Up'), ), const SizedBox(height: 16.0), Center( child: GestureDetector( onTap: _navigateToSignIn, child: RichText( text: const TextSpan( text: 'Have an account? ', style: TextStyle(color: Colors.black), children: <TextSpan>[ TextSpan( text: 'Sign In', style: TextStyle( fontWeight: FontWeight.bold, color: Colors.blue, ), ), ], ), ), ), ), const SizedBox(height: 16.0), const Center( child: Text('Or'), ), const SizedBox(height: 16.0), SignInButton( Buttons.Google, onPressed: () { // TODO: Implement Google sign-in logic here }, ), ], ), ), ), ), ); } // Goto SignUp Page void _navigateToSignIn() { Navigator.pushReplacement( context, MaterialPageRoute(builder: (context) => const SignIn()), ); } }
OUTPUT :
Conclusion
The sign-up page’s successful design lays the foundation for an engaging, user-centric onboarding experience in our Flutter ecommerce app. Through a straightforward, visually appealing sign-up process, we nurture a positive impression of our platform from the outset for users.
The next section delves into essential app development aspects: Firebase integration for authentication and data storage, product listing page design, user authentication, and user profile creation. Through our unwavering focus on user experience and the application of best practices, we progress toward crafting an exceptional Flutter ecommerce app that delights and captivates users.
Stay tuned for the upcoming sections as we continue our journey towards developing a fully functional and compelling ecommerce app using Flutter. Together, we’ll unlock the potential of Flutter and Firebase to deliver an exceptional user experience in our app.
Happy coding!
Click Here For More Related Bolgs!