Exploring Custom and Composite Widgets in Flutter
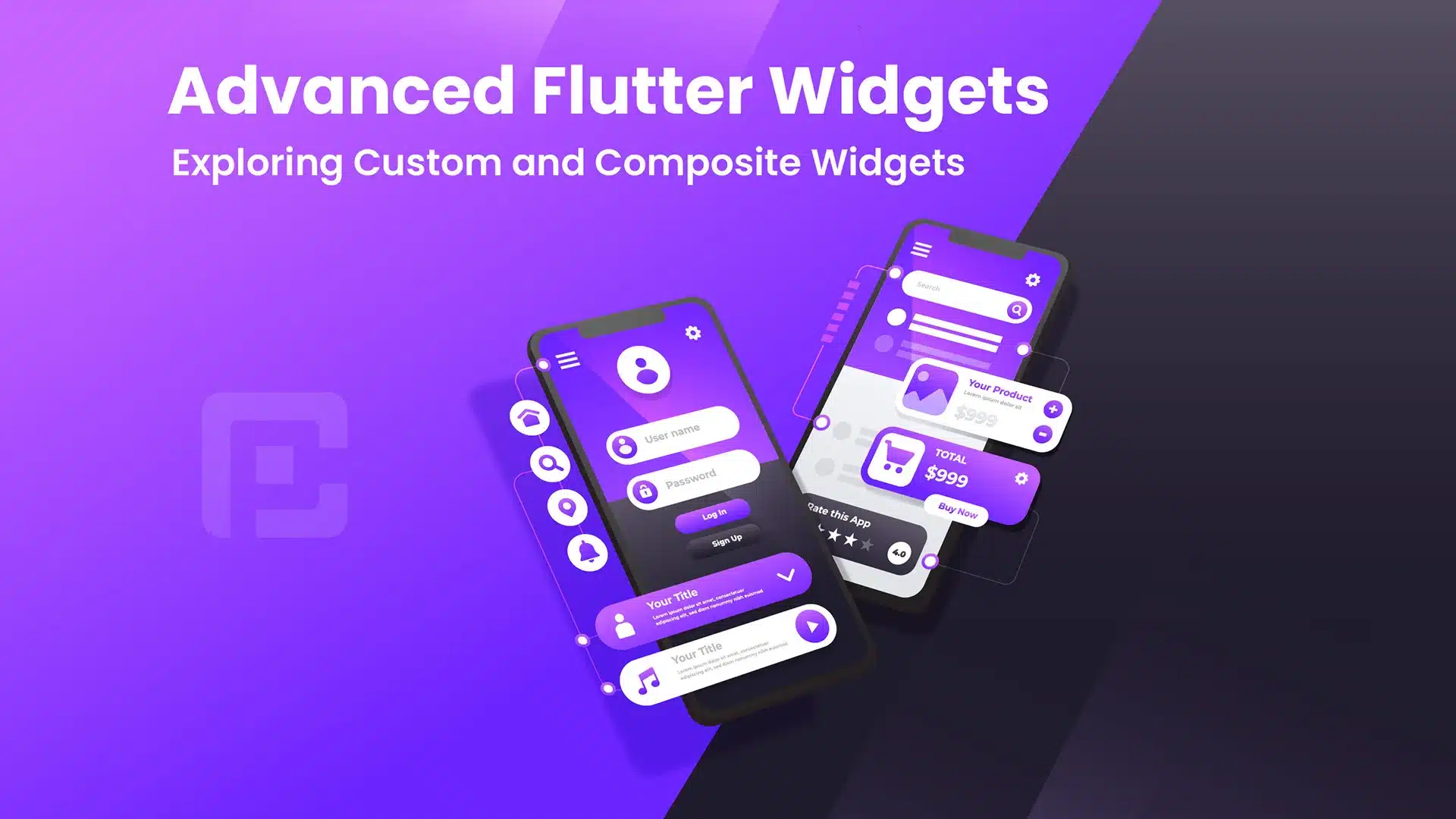
28 June
Flutter’s extensive widget library provides a solid foundation for building user interfaces, but there may come a time when you need to create more complex and customized UI elements. In this article, we will explore advanced Flutter widgets, specifically focusing on custom and composite widgets. By leveraging these powerful tools, you can create highly customizable and reusable components that cater to your app’s unique requirements. Join us on this journey as we delve into the world of advanced Flutter widgets with a practical code example.
Creating a Custom Button Widget
import 'package:flutter/material.dart'; class CustomButton extends StatelessWidget { final String text; final VoidCallback onPressed; const CustomButton({required this.text, required this.onPressed}); @override Widget build(BuildContext context) { return ElevatedButton( onPressed: onPressed, child: Text( text, style: TextStyle( fontSize: 16, fontWeight: FontWeight.bold, ), ), style: ElevatedButton.styleFrom( padding: EdgeInsets.symmetric(horizontal: 16, vertical: 10), primary: Colors.blue, onPrimary: Colors.white, shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(8), ), ), ); } }
In this code example, we create a custom button widget called CustomButton. It encapsulates the functionality and appearance of a button with customizable text and an onPressed callback. The button is styled with a blue background, white text, and rounded corners. By using this custom button widget, you can easily create buttons with consistent styling and behavior throughout your app.
Exploring Composite Widgets: Building a User Card
Composite widgets are widgets that combine multiple other widgets to create a more complex user interface element. Let’s take a look at an example of a composite widget that represents a user card:
import 'package:flutter/material.dart'; class UserCard extends StatelessWidget { final String name; final String email; final String avatarUrl; const UserCard({ required this.name, required this.email, required this.avatarUrl, }); @override Widget build(BuildContext context) { return Card( child: ListTile( leading: CircleAvatar( backgroundImage: NetworkImage(avatarUrl), ), title: Text(name), subtitle: Text(email), ), ); } }
In this example, the UserCard widget combines a Card widget with a ListTile to create a visually appealing user card. The card displays the user’s name, email, and an avatar image fetched from the provided URL. By using this composite widget, you can easily create user cards with consistent styling and layout.
Conclusion
Congratulations on exploring advanced Flutter widgets! By creating custom widgets and leveraging composite widgets, you can take your UI design and development skills to the next level. The code examples demonstrated the power and flexibility of custom and composite widgets, empowering you to build highly customized and reusable components in your Flutter applications. Don’t be afraid to experiment and create your own custom and composite widgets to cater to your app’s unique needs.
Happy coding!