Flutter vs React Native: Ease of Learning for Beginners with Examples
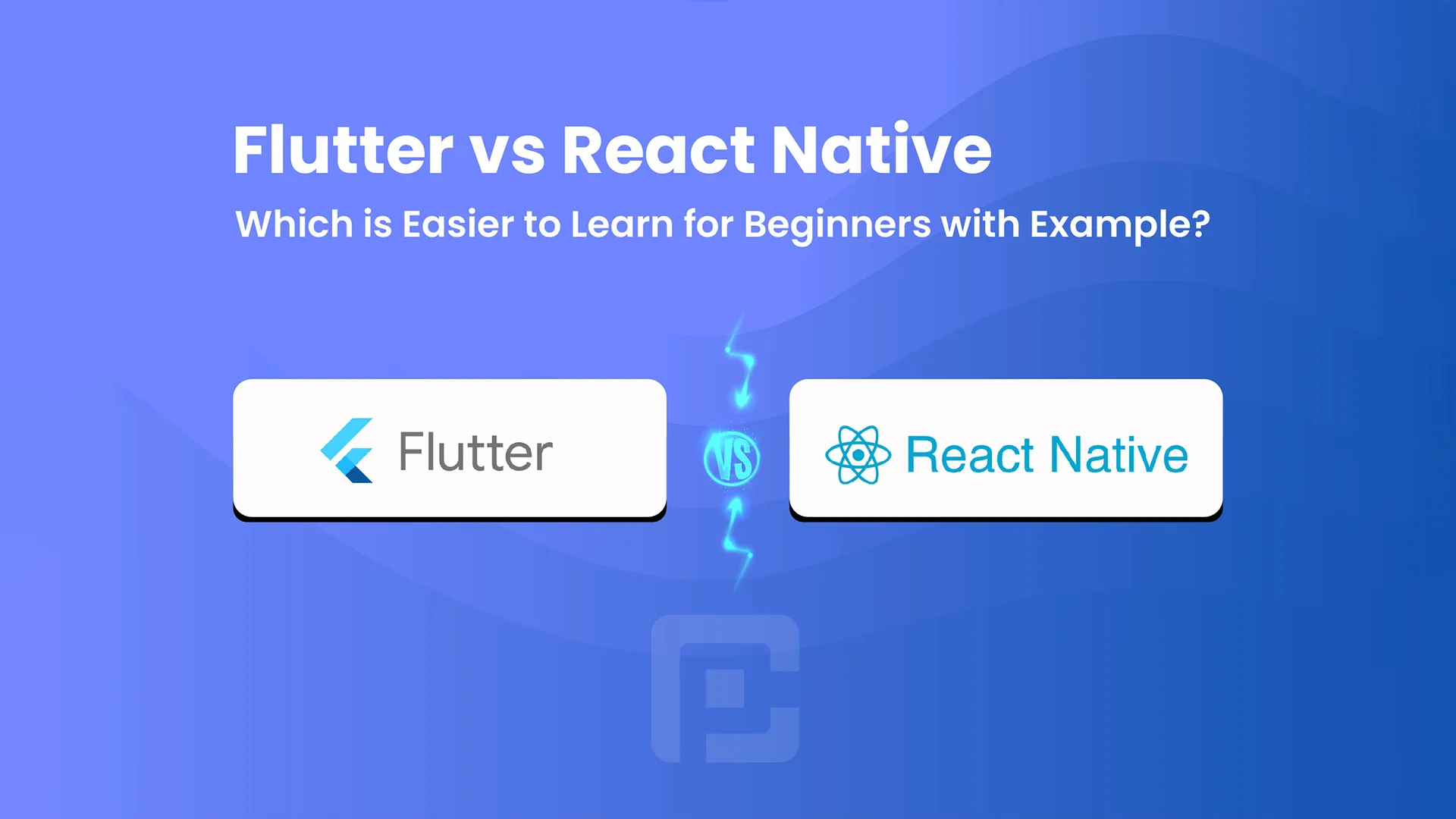
27 June
Flutter and React Native are two of the most popular frameworks for cross-platform app development. Both frameworks have their advantages and disadvantages, and developers often consider various factors when choosing between them. One of the most significant factors is ease of learning, especially for beginners. In this article, we will compare Flutter and React Native in terms of ease of learning for beginners with an example.
Flutter
Flutter, developed by Google, is a relatively new framework that has gained significant popularity since its release in 2017. Flutter uses the Dart programming language, which is easy to learn for developers who are familiar with object-oriented programming languages like Java or C#. Flutter’s reactive programming model and customizable widgets make it easy to create complex UIs and animations.
To illustrate how easy it is to learn Flutter, let’s consider a simple example of creating a button that changes its text when pressed. The code for this example in Flutter would look like this:
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { String buttonText = 'Press me!'; void _changeText() { setState(() { buttonText = 'You pressed me!'; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Flutter Demo'), ), body: Center( child: ElevatedButton( onPressed: _changeText, child: Text(buttonText), ), ), ); } }
In this example, we create a stateful widget, MyHomePage, that contains an ElevatedButton widget. When the button is pressed, the _changeText function is called, which changes the buttonText variable and triggers a rebuild of the widget. The Text widget inside the ElevatedButton widget displays the value of buttonText.
React Native
React Native, developed by Facebook, is a popular framework for cross-platform app development since its release in 2015. React Native uses JavaScript and JSX syntax, which is easy to learn for developers who are familiar with web development. React Native’s reusable components and virtual DOM make it easy to create high-performance apps with a native look and feel.
To illustrate how easy it is to learn React Native, let’s consider the same example of creating a button that changes its text when pressed. The code for this example in React Native would look like this:
import React, { useState } from 'react'; import { StyleSheet, Text, View, Button } from 'react-native'; export default function App() { const [buttonText, setButtonText] = useState('Press me!'); const changeText = () => { setButtonText('You pressed me!'); }; return ( <View style={styles.container}> <Text style={styles.text}>React Native Demo</Text> <Button title={buttonText} onPress={changeText} /> </View> ); } const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#fff', alignItems: 'center', justifyContent: 'center', }, text: { fontSize: 20, fontWeight: 'bold', marginBottom: 20, }, });
In this example, we create a functional component, App, that contains a Button component. When the button is pressed, the changeText function is called, which updates the buttonText variable using the setButtonText function. The Button component displays the value of buttonText.
Conclusion
In conclusion, both Flutter and React Native are easy to learn for beginners, and both frameworks have their advantages and disadvantages. Flutter uses the Dart programming language and customizable widgets, while React Native uses JavaScript and JSX syntax and reusable components. Both frameworks have excellent documentation and resources for beginners, making it easy to get started with cross-platform app development. Ultimately, the choice between Flutter and React Native will depend on the developer’s specific needs and preferences.