How to Build a Flutter App with Machine Learning
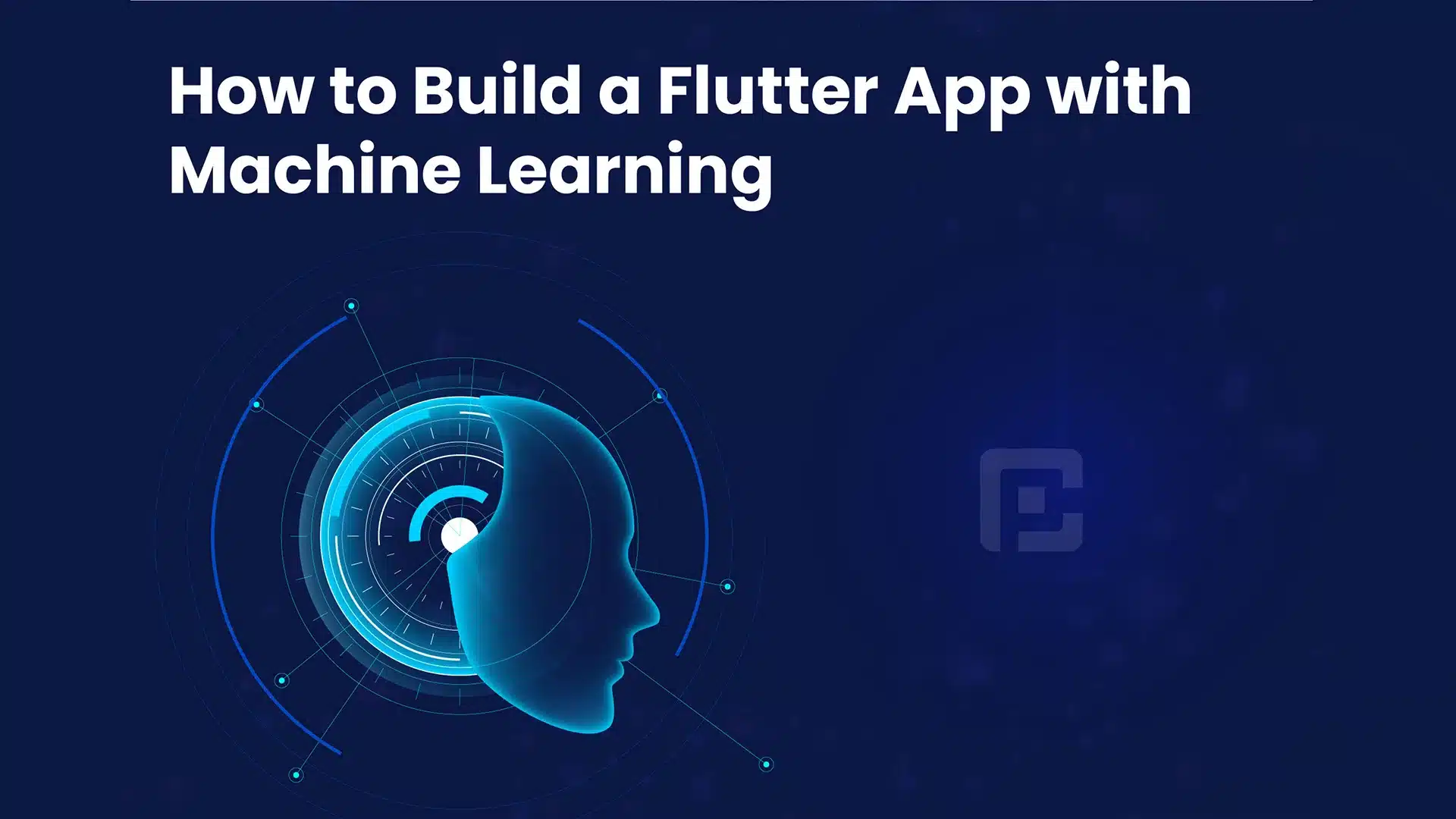
29 June
Machine learning is a powerful technology that can be used to create apps that can learn and adapt to their users. Flutter is a cross-platform mobile development framework that can be used to build beautiful, high-performance apps for Android, iOS, web, and desktop.
In this article, we will show you how to build a Flutter app with machine learning. We will use the TensorFlow Lite: https://www.tensorflow.org/lite/ framework to train a machine learning model and then use that model in our Flutter app.
Prerequisites
To follow this tutorial, you will need the following:
- A computer with Flutter installed
- A basic understanding of Flutter
- A basic understanding of machine learning
Step 1: Create a Flutter project
The first step is to create a new Flutter project. You can do this by running the following command in your terminal:
flutter create my_app
This will create a new directory called my_app with all the necessary files for your Flutter project.
Step 2: Train a machine learning model
In this step, we will train a machine learning model. We will use the TensorFlow Lite: https://www.tensorflow.org/lite/ framework to train a simple model that can classify images of cats and dogs.
To train the model, you will need to have a dataset of images of cats and dogs. You can find a dataset of images online, or you can create your own.
Once you have a dataset of images, you can train the model by running the following command in your terminal:
tensorflow lite train --model_path=my_model.tflite --data_path=my_dataset
This will train the model and save it to the file my_model.tflite.
Step 3: Use the machine learning model in your Flutter app
In this step, we will use the machine learning model in our Flutter app. We will create a simple app that can classify images of cats and dogs.
To use the machine learning model in your Flutter app, you will need to import the tflite package. You can do this by adding the following line to your pubspec.yaml file:
dependencies: tflite: ^1.1.2
Once you have imported the tflite package, you can use the loadModel function to load the machine learning model into your app. You can then use the classifyImage function to classify an image.
The following code shows how to use the tflite package to classify an image:
import 'package:tflite/tflite.dart'; void main() { // Load the machine learning model. var model = await loadModel('my_model.tflite'); // Classify an image. var image = await getImage(); var classification = classifyImage(image, model); // Display the classification. print(classification); }
This code will load the machine learning model from the file my_model.tflite and then classify an image. The classification will be printed to the console.
Conclusion
In this article, we showed you how to build a Flutter app with machine learning. We used the TensorFlow Lite framework to train a simple model that can classify images of cats and dogs. We then used the machine learning model in our Flutter app to classify images.
You can use the same steps to build your own Flutter apps with machine learning. There are many different machine learning models that you can use, and there are many different ways to use them in your apps. With a little creativity, you can build amazing apps that use machine learning to learn and adapt to their users.