Develop APIs in Minutes with Laravel Orion
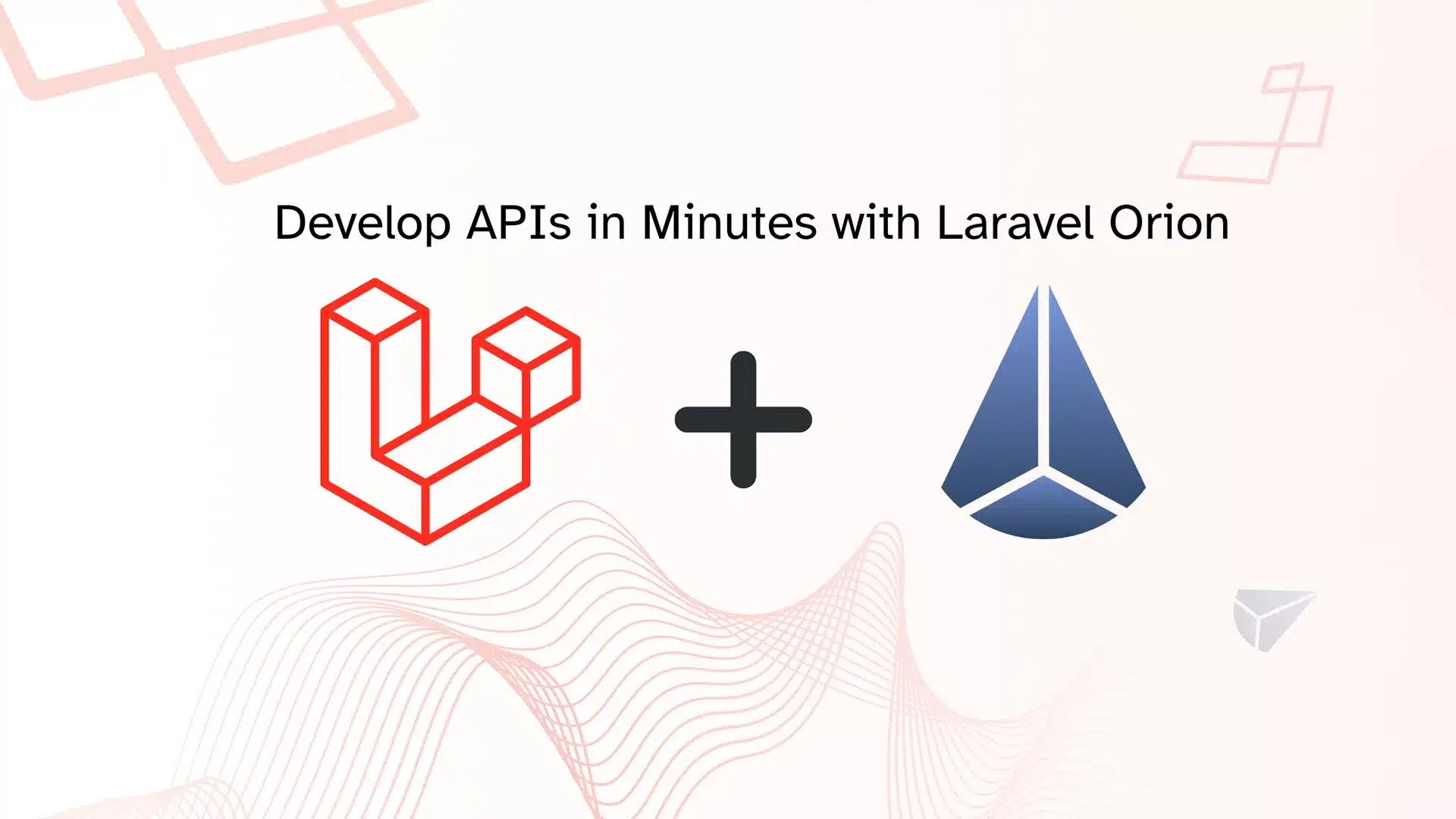
1 September
A Step-by-Step Guide
Are you ready to take your API development skills to the next level? Look no further! In this blog post, we will introduce you to Laravel Orion, a powerful API toolkit that will change the way you build APIs with Laravel framework. The simplest way to create REST API with Laravel.
What is Laravel Orion?
Laravel Orion is a package that simplifies the process of building RESTful APIs in Laravel. It provides a set of functionalities and conventions that help you structure and organize your API code while following best practices.
With Laravel Orion, you can easily define API resources, handle CRUD operations, and even manage complex relationships between resources. It streamlines the development process and allows you to focus on what matters most: building a robust API for your application.
Getting Started
To get started with Laravel Orion, make sure you have a Laravel project up and running. If you haven’t installed Laravel, head over to the official Laravel website for installation instructions.
Once your project is set up, you can install Laravel Orion using Composer:
composer require tailflow/laravel-orion
After successful installation, you will need to publish the Laravel Orion configuration file. This file allows you to customize the behavior of Laravel Orion:
php artisan vendor:publish --tag=orion-config
Defining API Resources
With Laravel Orion, defining your API resources is a breeze. Let’s say we want to build an API for managing products in an e-commerce application. Let’s begin by creating a Product model and a corresponding resource for it.
php artisan make:model Product
CRUD Operations
Once you have defined your API resources, Laravel Orion provides a full suite of CRUD operations out of the box.
Sample Code
Now that we have covered the basic concepts of Laravel Orion, here’s a simple example of how you can use it to build an e-commerce API:
<?php namespace App\Http\Controllers; use App\Http\Resources\ProductResource; use App\Models\Product; use Tailflow\Orion\Http\Controllers\OrionController; class ProductsController extends OrionController { protected $model = Product::class; // or "App\Models\Product" }
In this example, we create a ProductController that extends the OrionController provided by Laravel Orion. We specify the controller to use the Product model class.
Finally, register the route in api.php by calling Orion::resource
<?php use Illuminate\Support\Facades\Route; use Orion\Facades\Orion; use App\Http\Controllers\ProductsController; Route::group(['as' => 'api.'], function() { Orion::resource(products, ProductsController::class); });
We now have a fully functioning API for managing products. You can test the API using tools like Postman or any REST client of your choice.
+--------+-----------+----------------------+---------------------------+----------------------------------------------------------+------------+ | Domain | Method | URI | Name | Action | Middleware | +--------+-----------+----------------------+---------------------------+----------------------------------------------------------+------------+ ... | | GET|HEAD | api/products | api.products.index | App\Http\Controllers\Api\ProductsController@index | api | | | POST | api/products/search | api.products.search | App\Http\Controllers\Api\ProductsController@index | api | | | POST | api/products | api.products.store | App\Http\Controllers\Api\ProductsController@store | api | | | GET|HEAD | api/products/{post} | api.products.show | App\Http\Controllers\Api\ProductsController@show | api | | | PUT|PATCH | api/products/{post} | api.products.update | App\Http\Controllers\Api\ProductsController@update | api | | | DELETE | api/products/{post} | api.products.destroy | App\Http\Controllers\Api\ProductsController@destroy | api | | | POST | api/products/batch | api.products.batchStore | App\Http\Controllers\Api\ProductsController@batchStore | api | | | PATCH | api/products/batch | api.products.batchUpdate | App\Http\Controllers\Api\ProductsController@batchUpdate | api | | | DELETE | api/products/batch | api.products.batchDestroy | App\Http\Controllers\Api\ProductsController@batchDestroy | api |
Conclusion
Congratulations! You have just scratched the surface of what Laravel Orion has to offer. In this blog post, we introduced Laravel Orion and demonstrated how to define API resources and perform CRUD operations.
Stay tuned for our upcoming blog series: “Building an E-Commerce API with Laravel Orion.” In this series, we will dive deeper into Laravel Orion and explore more advanced topics, such as handling relationships, authentication, and pagination.
Happy coding!
Click Here for the related Blogs!