Creating CRUD application with React JS
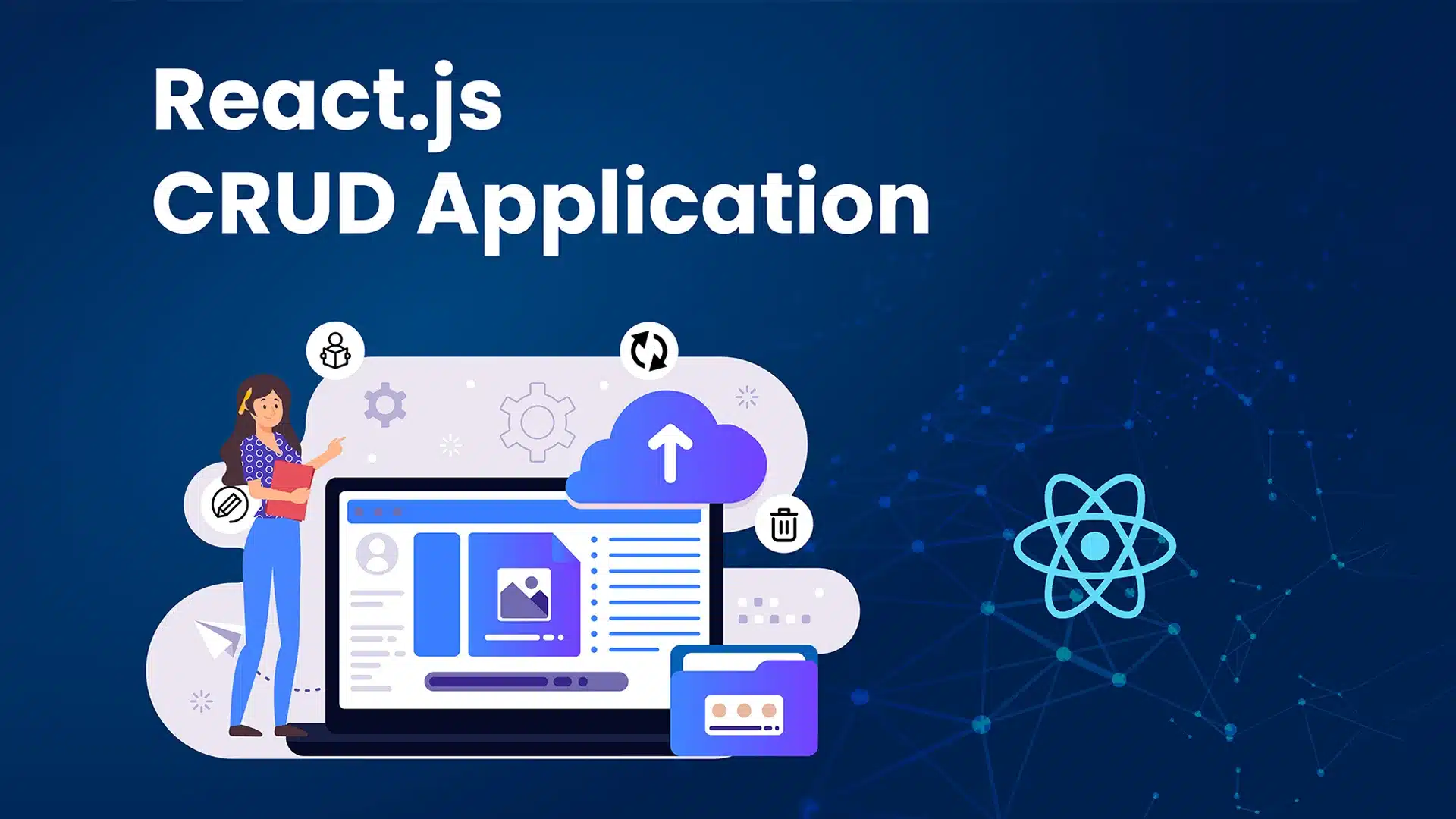
28 April
Making use of Crud in React JS: A step by step creation
CRUD operations play a vital role in enabling web applications to interact with data. Using React JS, CRUD operations help users in executing the most fundamental data management tasks, such as generating new records, retrieving existing records, modifying or updating records, and deleting records. These operations enable web applications to function smoothly and efficiently, while providing users with seamless access to data. In essence, CRUD operations accomplished with a built in React JS, act as the cornerstone of web application development and form the basis of most data-driven applications.
Why use Crud in React JS?
CRUD operations are fundamental to any application to manage data. In React, CRUD operations are essential to building dynamic user interfaces and providing a seamless user experience. Here are the reasons why CRUD is important in React:
- Creating new data: This typically involves collecting user input through a form or dialog box and then submitting that data to the server. With CRUD operations, developers can easily create the necessary UI components and event handlers to manage the data creation process.
- Reading data: This typically involves retrieving data from the server and displaying it in the UI. With CRUD operations, developers can create components to display the data in various ways, such as tables, lists, or charts.
- Updating data: This typically involves creating UI components to display the data for editing and then submitting those changes back to the server. With CRUD operations, developers can create the necessary components and event handlers to manage data updates in a structured and consistent way.
Updating data: This typically involves creating UI components to display the data for editing and then submitting those changes back to the server. With CRUD operations, developers can create the necessary components and event handlers to manage data updates in a structured and consistent way.
Building Crud in React through a Step-by-Step Approach
Here is a step-by-step guide to building a CRUD application in React:
- Set up the project: Start by creating a new React project using a tool like Create React App. This will generate a basic project structure with a few files and folders.
- Define the data model: Decide on the data model for your CRUD application. This will determine what data to store and how it should be structured. For example, if building a simple to-do list application, a data model might consist of tasks with a name and completion status.
- Create the component structure: Create a set of React components to represent different parts of a CRUD application. This might include a component to display the list of items, a component to create new items, a component to edit existing items, and a component to delete items.
- Manage state: Use the useState hook to manage state in components. This allows to keep track of the current list of items, the item being edited or deleted, and any changes made to the item data.
- Add user interactions: Add event handlers to components to handle user interactions, such as clicking a button to add a new item, clicking a button to edit an existing item, or clicking a button to delete an item.
- Update the UI: Use React’s virtual DOM and state management to efficiently update the UI when the user interacts with the application. This might involve re-rendering the entire list of items when a new item is added or updating only the specific item being edited when changes are made.
Creating Crud in React with Practical Examples
Let us understand the concept of Crud more closely by looking at a couple of basic examples of creating a CRUD (Create, Read, Update, Delete) application in React JS.
Example 1
Let us assume we want to create a simple application allowing us to manage a list of users.
First, we will create a new React component called Users. This component will render a list of users and allow us perform CRUD operations on them.
import React, { useState } from 'react'; function Users() { const [users, setUsers] = useState([]); const handleAddUser = (user) => { setUsers([...users, user]); }; const handleDeleteUser = (index) => { setUsers(users.filter((_, i) => i !== index)); }; const handleUpdateUser = (index, user) => { setUsers([ ...users.slice(0, index), user, ...users.slice(index + 1) ]); }; return ( <div> <h1>Users</h1> <UserForm onAddUser={handleAddUser} /> <UserList users={users} onDeleteUser={handleDeleteUser} onUpdateUser={handleUpdateUser} /> </div> ); } export default Users;
In this component, we are using the useState hook to manage the state of our users list. We’re also defining three functions to handle the CRUD operations: handleAddUser, handleDeleteUser, and handleUpdateUser.
Next, we create two new components called UserForm and UserList. The UserForm component will render a form allowing us to add new users, while the UserList component will render a list of existing users and allow us to delete or update them.
import React, { useState } from 'react'; function UserForm({ onAddUser }) { const [name, setName] = useState(''); const [email, setEmail] = useState(''); const handleSubmit = (event) => { event.preventDefault(); onAddUser({ name, email }); setName(''); setEmail(''); }; return ( <form onSubmit={handleSubmit}> <input type="text" placeholder="Name" value={name} onChange={(event) => setName(event.target.value)} /> <input type="email" placeholder="Email" value={email} onChange={(event) => setEmail(event.target.value)} /> <button type="submit">Add User</button> </form> ); } function UserList({ users, onDeleteUser, onUpdateUser }) { return ( <ul> {users.map((user, index) => ( <li key={index}> {user.name} ({user.email}) <button onClick={() => onDeleteUser(index)}>Delete</button> <button onClick={() => onUpdateUser(index, user)}>Edit</button> </li> ))} </ul> ); } export { UserForm, UserList };
In the UserForm component, we are using the useState hook to manage the state of our form inputs. When the form is submitted, we call the onAddUser function with the new user object and reset the form inputs. In the UserList component, we’re rendering a list of users and defining two buttons to delete or update each user.
When the delete button is clicked, we call the onDeleteUser function with the index of the user to be deleted. When the edit button is clicked, we call the onUpdateUser function with the index and current user object, and pass it to a new component called UserEditForm, which will render a form with the current user data and allow us to update it.
import React, { useState } from 'react'; function UserEditForm({ user, onUpdateUser, onCancel }) { const [name, setName] = useState(user.name); const [email, setEmail] = useState(user.email); const handleSubmit = (event) => { event.preventDefault(); onUpdateUser({ ...user, name, email }); }; return ( <form onSubmit={handleSubmit}> <input type="text" placeholder="Name" value={name} onChange={(event) => setName(event.target.value)} /> <input type="email" placeholder="Email" value={email} onChange={(event) => setEmail(event.target.value)} /> <button type="submit">Update</button> <button type="button" onClick={onCancel}>Cancel</button> </form> ); } export default UserEditForm;
Finally, we will update the handleUpdateUser function in the Users component to render the UserEditForm component when the edit button is clicked, and allow us to update the user data.
import React, { useState } from 'react'; import { UserForm, UserList } from './UserComponents'; import UserEditForm from './UserEditForm'; function Users() { const [users, setUsers] = useState([]); const [editUser, setEditUser] = useState(null); const handleAddUser = (user) => { setUsers([...users, user]); }; const handleDeleteUser = (index) => { setUsers(users.filter((_, i) => i !== index)); }; const handleUpdateUser = (index, user) => { setEditUser(user); setUsers(users.filter((_, i) => i !== index)); }; const handleCancelUpdate = () => { setEditUser(null); }; const handleSaveUpdate = (user) => { setUsers([...users, user]); setEditUser(null); }; return ( <div> <h1>Users</h1> {editUser ? ( <UserEditForm user={editUser} onUpdateUser={handleSaveUpdate} onCancel={handleCancelUpdate} /> ) : ( <UserForm onAddUser={handleAddUser} /> ) } <UserList users={users} onDeleteUser={handleDeleteUser} onUpdateUser={handleUpdateUser} /> </div> ); } export default Users;
In this updated handleUpdateUser function, we are setting the editUser state to the current user object and removing it from the users array. We are also defining two new functions to handle the cancel and save actions of the UserEditForm. When the editUser state is not null, we’re rendering the UserEditForm component instead of the UserForm.
Example 2
Let us create a CRUD application in React JS using functional components and hooks:
import React, { useState } from 'react'; function Crud() { const [items, setItems] = useState([ { id: 1, name: 'Item 1', description: 'This is the first item' }, { id: 2, name: 'Item 2', description: 'This is the second item' }, { id: 3, name: 'Item 3', description: 'This is the third item' }, ]); const [editingItem, setEditingItem] = useState(null); const handleInputChange = (event) => { const { name, value } = event.target; setEditingItem((prevEditingItem) => ({ ...prevEditingItem, [name]: value, })); }; const handleAddItem = (event) => { event.preventDefault(); const newItem = { id: Date.now(), name: '', description: '' }; setItems([...items, newItem]); setEditingItem(newItem); }; const handleEditItem = (item) => { setEditingItem(item); }; const handleDeleteItem = (item) => { setItems(items.filter((i) => i.id !== item.id)); setEditingItem(null); }; const handleSaveItem = (event) => { event.preventDefault(); if (!editingItem.name || !editingItem.description) { return; } const newItems = items.map((item) => item.id === editingItem.id ? editingItem : item ); setItems(newItems); setEditingItem(null); }; return ( <div> <h1>CRUD Example</h1> <ul> {items.map((item) => ( <li key={item.id}> {editingItem === item ? ( <form onSubmit={handleSaveItem}> <input type="text" name="name" value={editingItem.name} onChange={handleInputChange} /> <input type="text" name="description" value={editingItem.description} onChange={handleInputChange} /> <button type="submit">Save</button> <button type="button" onClick={() => setEditingItem(null)}> Cancel </button> </form> ) : ( <div> <h2>{item.name}</h2> <p>{item.description}</p> <button onClick={() => handleEditItem(item)}>Edit</button> <button onClick={() => handleDeleteItem(item)}>Delete</button> </div> ) } </li> ))} </ul> <button onClick={handleAddItem}>Add Item</button> </div> ); } export default Crud;
In this example, we are using the useState hook to manage the items and editingItem states. The items state is an array of objects representing the items in the list, and the editingItem state is an object representing the item currently being edited.
We are using the handleInputChange function to update the editingItem state when the user enters data into the input fields. We are also using the handleAddItem, handleEditItem, and handleDeleteItem functions to add, edit, and delete items in the list. Finally, we are using the handleSaveItem function to save changes made to an item in the list.
Summing Up
Overall, CRUD is essential to build dynamic and interactive applications in React. Developers can create a consistent and predictable user experience, while ensuring data is managed safely and securely.