React JS With Docker
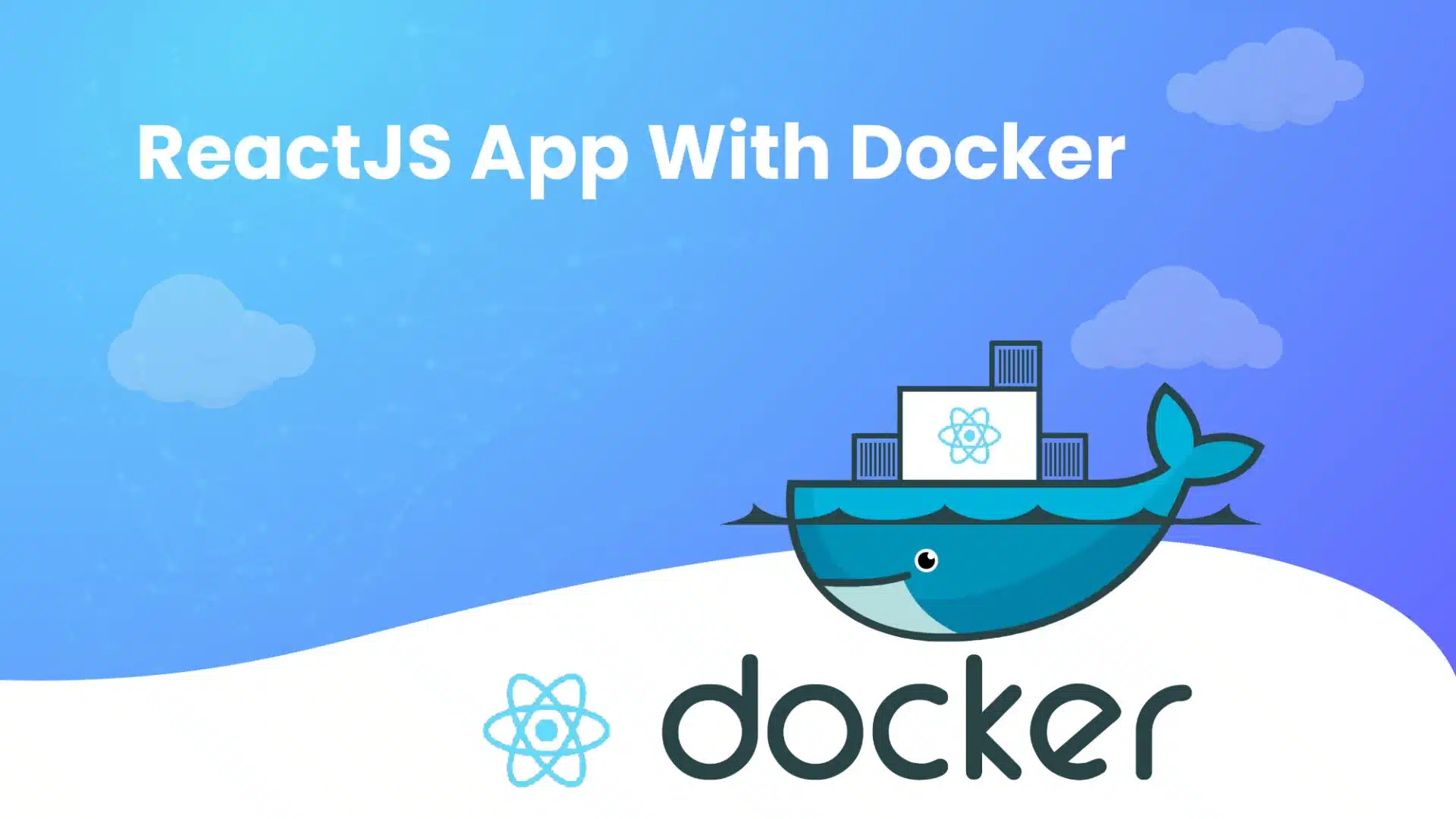
28 April
Creating a ReactJS application with Docker – All you need to know
ReactJS is a popular JavaScript library used for building dynamic and interactive user interfaces. Docker is a popular platform that simplifies the deployment and management of applications. Combining ReactJS with Docker allows developers to easily create, manage, and deploy their React applications.
Here is a step-by-step guide on how to set up ReactJS with Docker:
Step 1: Create a new ReactJS project Create a new ReactJS project using the create-react-app CLI tool. Open a terminal and run the following command:
npx create-react-app my-app
Step 2: Create a Dockerfile In order to build a Docker image for the ReactJS application, we need to create a Dockerfile. The Dockerfile describes the image that we want to build. Create a new file called Dockerfile in the root directory of your ReactJS project and add the following contents:
FROM node:14-alpine
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD [“npm”, “start”]
This Dockerfile specifies that the image should be based on the official Node.js 14 Alpine image. It also sets the working directory to /app, copies the package.json and package-lock.json files, installs the dependencies, copies the rest of the application code, exposes port 3000, and starts the application using the npm start command.
Step 3: Build the Docker image To build the Docker image, navigate to the root directory of your ReactJS project in a terminal and run the following command:
docker build -t my-react-app.
This command builds a Docker image with the name my-react-app, using the Dockerfile in the current directory.
Step 4: Run the Docker container Once the Docker image is built, we can run the Docker container using the following command:
docker run -p 3000:3000 my-react-app
This command runs the Docker container with the name my-react-app and maps port 3000 on the host machine to port 3000 in the Docker container.
Step 5: Access the ReactJS application Finally, open a web browser and navigate to http://localhost:3000 to access the ReactJS application running inside the Docker container.
Setting up ReactJS with Docker involves creating a Dockerfile, building a Docker image, and running a Docker container. Docker do simplify the deployment and management of ReactJS applications with a consistent and isolated environment.
Using Docker to Setup a ReactJS Application
We will look at a couple of ways to set up a React JS application with Docker can be a great way to ensure that your development environment is consistent across multiple machines and easily portable.
Method 1
This method uses the default port of create-react-app, which is 3000, and exposes it to the host machine using the EXPOSE command in the Dockerfile. The app is run with the “npm start” command, which starts the development server. The Dockerfile simply copies the app files to the container, installs any necessary dependencies, and runs the app.
- Create a new React JS app using create-react-app by running the following command in your terminal:
npx create-react-app my-app
- Change your current working directory to the newly created app directory by running the following command:
cd my-app
- Create a new Dockerfile in the root directory of your app by running the following command:
ouch Dockerfilet
- Open the Dockerfile in your preferred text editor and add the following code:
# Use an official Node.js runtime as a parent image
FROM node:14-alpine
# Set the working directory to /app
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages
RUN npm install
# Make port 3000 available to the world outside this container
EXPOSE 3000
# Run app.js when the container launches
CMD [“npm”, “start”]
This Dockerfile uses the official Node.js Docker image, sets the working directory to /app, copies the current directory (i.e., your React app) into the container, installs any needed packages with npm install, exposes port 3000 (the default port used by create-react-app), and runs the app with npm start.
- Build a Docker image by running the following command:
docker build -t my-app.
This command will build a Docker image with the name my-app using the Dockerfile in the current directory.
- Once the image is built, you can run a container with the following command:
docker run -it –rm -p 3000:3000 my-app
This command will start a container based on the my-app image, open port 3000 on the host machine (i.e., your computer) and map it to port 3000 inside the container, and remove the container automatically when you stop it (–rm option).
- Now you should be able to view your React app by opening a web browser and navigating to http://localhost:3000.
This is a simpler method intended for development purposes.
Method 2
This method builds the React app using the “npm run build” command and serves it using the “serve” package. The app is served on port 80 and this port is exposed to the host machine using the EXPOSE command in the Dockerfile. This method copies both the package.json and package-lock.json files to the container and installs the dependencies using the “npm install” command. Then it copies the rest of the app files to the container and builds the app before running it using the “serve” command.
- Create a new React JS app using create-react-app by running the following command in your terminal:
npx create-react-app my-app
- Change your current working directory to the newly created app directory by running the following command:
cd my-app
- Create a new Dockerfile in the root directory of your app by running the following command:
touch Dockerfile
- Open the Dockerfile in your preferred text editor and add the following code:
# Use an official Node.js runtime as a parent image
FROM node:14-alpine
# Set the working directory to /app
WORKDIR /app
# Copy the package.json and package-lock.json files to the container
COPY package*.json ./
# Install any needed packages
RUN npm install
# Copy the rest of the application files to the container
COPY . .
# Build the application
RUN npm run build
# Make port 80 available to the world outside this container
EXPOSE 80
# Run the application with serve
CMD [“npx”, “serve”, “-s”, “build”, “-l”, “80”]
This Dockerfile uses the official Node.js Docker image, sets the working directory to /app, copies the package.json and package-lock.json files to the container, installs any needed packages with npm install, copies the rest of the application files to the container, builds the application with npm run build, exposes port 80, and runs the application with the serve package.
-
- Build a Docker image by running the following command:
This command will build a Docker image with the name my-app using the Dockerfile in the current directory.
-
- Once the image is built, you can run a container with the following command:
This command will start a container based on the my-app image, open port 80 on the host machine (i.e., your computer) and map it to port 80 inside the container, and remove the container automatically when you stop it (–rm option).
- Now you should be able to view your React app by opening a web browser and navigating to http://localhost.
- This method is more suitable for production environments because it builds and serves a more optimized version of the app.
Last Few Words to Sum Up
We looked at a couple of ways to set up a ReactJS application with Docker. However, with more knowledge on this, one can set up ReactJS with Docker in a few other ways as well. Not to mention, it does offer several benefits for developers. Here are some of the reasons why developers may choose to use Docker for their ReactJS projects:
- Consistency: Docker provides a consistent environment for developing and deploying ReactJS applications. Developers can be sure that their application will work the same way across different environments, making it easier to deploy and manage their application.
- Isolation: Docker allows for isolation between the application and the host machine. This means that the application is less likely to be affected by changes to the host system, making it more stable and reliable.
- Scalability: Docker makes it easy to scale up or down the application based on demand. Developers can quickly spin up new containers to handle increased traffic or scale down when traffic decreases, without having to worry about the underlying infrastructure.
- Portability: Docker containers can be easily moved between different environments, making it easier to deploy the application to different hosting providers or cloud platforms.
- Packaging: Docker allows developers to package the application and its dependencies into a single image, making it easier to share and deploy the application across different environments.
Using Docker for ReactJS projects provides a consistent, isolated, scalable, portable, and efficient environment for developing and deploying applications.
Method 1 is simpler and is intended for development purposes, while Method 2 is more suitable for production environments because it builds and serves a more optimized version of the app.