Design Forgot Password Page in Flutter Ecommerce
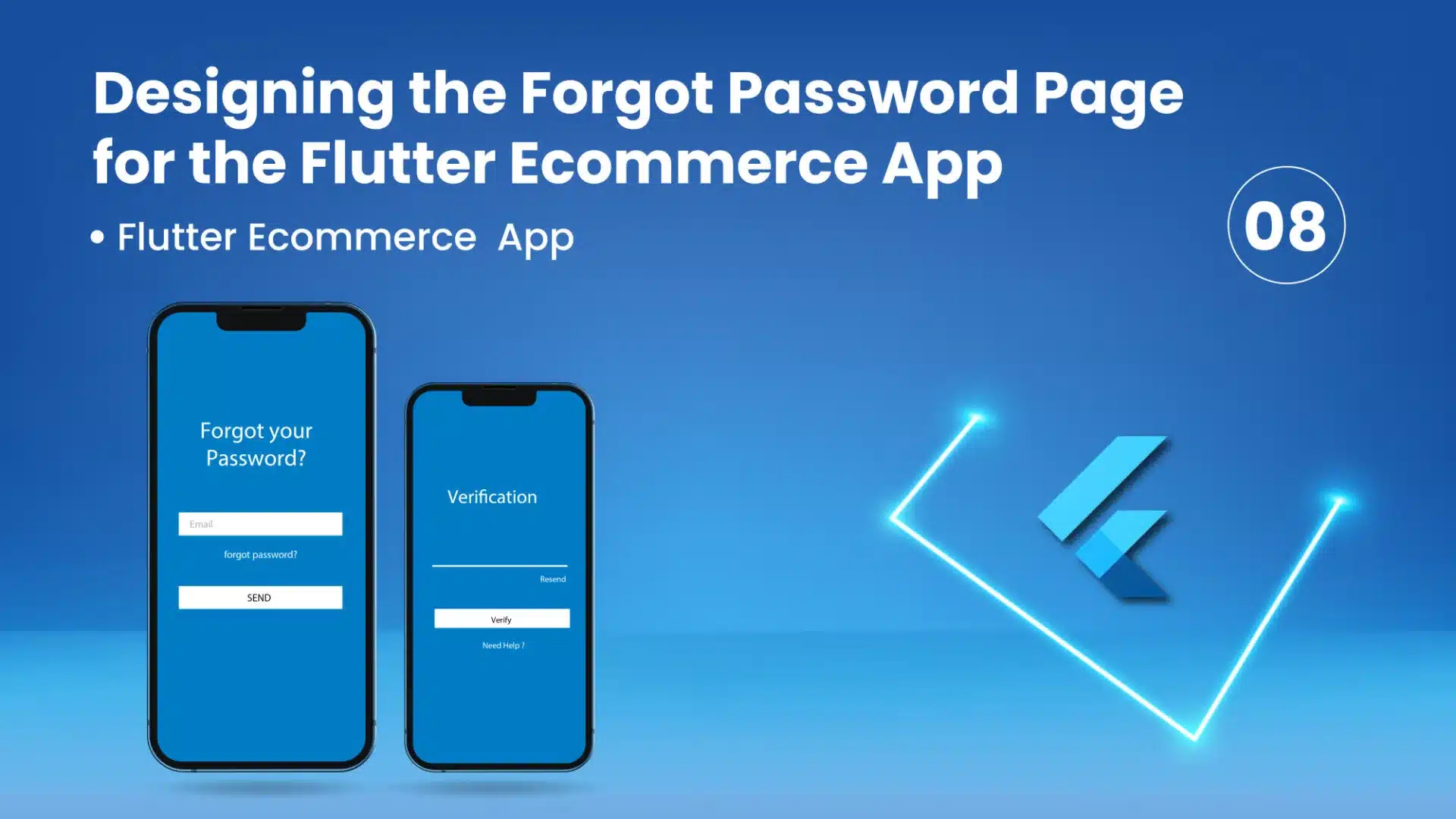
27 July
In this section, we will focus on design the forgot password page for our Flutter ecommerce app. The forgot password page allows users to reset their forgotten passwords and regain access to their accounts. We will design a simple and user-friendly forgot password page that includes an email input field for users to enter their registered email address. Additionally, we will add a submit button to initiate the password reset process. By following the principles of responsive design, we will ensure that the page layout is optimized for various screen sizes. With an effective and visually appealing forgot password page, we aim to provide a hassle-free and secure password recovery experience for our app users. Let’s proceed with designing the forgot password page to offer a seamless account recovery option in our app.
import 'package:clickmart/view/authentication/sign_in.dart'; import 'package:flutter/material.dart'; class ForgotPassword extends StatefulWidget { const ForgotPassword({super.key}); @override State<ForgotPassword> createState() => _ForgotPasswordState(); } class _ForgotPasswordState extends State<ForgotPassword> { // Form Key final GlobalKey<FormState> _formKey = GlobalKey<FormState>(); // TextForm Controller TextEditingController emailController = TextEditingController(); // Form Validation bool _validateAndSave() { final form = _formKey.currentState; if (form!.validate()) { form.save(); return true; } return false; } void _validateAndSubmit() { if (_validateAndSave()) { // TODO: Perform sign-up logic here } } @override Widget build(BuildContext context) { return WillPopScope( onWillPop: () { return Future.value(false); }, child: Scaffold( appBar: AppBar( automaticallyImplyLeading: false, title: const Text('Forgot Password'), ), body: Container( padding: const EdgeInsets.all(16.0), child: Form( key: _formKey, child: Column( crossAxisAlignment: CrossAxisAlignment.stretch, children: <Widget>[ TextFormField( controller: emailController, decoration: const InputDecoration(labelText: 'Email'), validator: (value) { if (value!.isEmpty) { return 'Please enter your email'; } return null; }, onSaved: (value) => emailController.text = value!, ), const SizedBox(height: 16.0), ElevatedButton( onPressed: _validateAndSubmit, child: const Text('Submit'), ), const SizedBox(height: 16.0), Center( child: GestureDetector( onTap: _navigateToSignIn, child: RichText( text: const TextSpan( text: 'Have an account? ', style: TextStyle(color: Colors.black), children: <TextSpan>[ TextSpan( text: 'Sign In', style: TextStyle( fontWeight: FontWeight.bold, color: Colors.blue, ), ), ], ), ), ), ), ], ), ), ), ), ); } // Goto SignUp Page void _navigateToSignIn() { Navigator.pushReplacement( context, MaterialPageRoute(builder: (context) => const SignIn()), ); } }
Here is the expected output screenshot of aim to design the forgot password page for our Flutter ecommerce app.
OUTPUT :